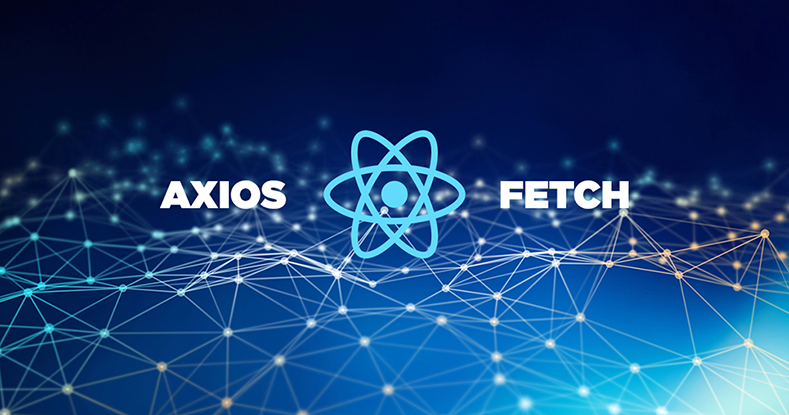
Introduction
We know that only API can separate the frontend from the backend. This tutorial will show the typical scenario of how to properly call Axios and Fetch with API in react application and how it works.
Before Starting its recommended to you have basic knowledge on ReactJS like create-react-app, functional components, class components, JSX, props and state, useState and useEffect hooks, setState, component lifecycle methods, conditional rendering, list, and keys, building simple forms, and of course necessary JavaScript especially ES6 syntaxes.
In this Article
- Introduction
- Rest API
- Axios
- Fetch API
- Fetch API in React
- Axios in React
- Conclusion
- Resouces/Further Reading
Rest API
The REST API(Representational State Transfer) is built on REST architecture, and it has six constraints. Initially communicated by Roy Fielding. It’s not a language or platform-specific, but it can communicate and create an interface with any other language or platform.
These six constraints are:
- Uniform Interface
- Stateless
- Cacheable
- Client-Server
- Layered System
- Code on Demand
Here is an example of REST API https://jsonplaceholder.typicode.com/posts, a fake online REST API for testing and prototyping.
In this tutorial, we would like to work on NewsApi to fetch news and get it into our application. We have to register individually to get the API key. If you haven’t one, just register for one!
NewsAPI Example
{ "status": "ok", "totalResults": 10, "articles": [ { "source": { "id": "techcrunch", "name": "TechCrunch" }, "author": "Jonathan Shieber", "title": "Impossible Foods nabs some Canadian fast food franchises as it expands in North America", "description": "After rolling out in some of Canada’s most high-falutin burger bistros, Impossible Foods is hitting Canada’s fast casual market with new menu items at national chains like White Spot and Triple O’s, Cactus Club Cafe, and Burger Priest. While none of those nam…", "url": "https://techcrunch.com/2020/09/21/impossible-foods-nabs-some-canadian-fast-food-franchises-as-it-expands-in-north-america/", "urlToImage": "https://techcrunch.com/wp-content/uploads/2020/08/GettyImages-1192592513.jpeg?w=601", "publishedAt": "2020-09-21T22:36:45Z", "content": "After rolling out in some of Canada’s most high-falutin burger bistros, Impossible Foods is hitting Canada’s fast casual market with new menu items at national chains like White Spot and Triple O’s, … [+671 chars]" }, ] }
You can implement any API you would like to use. Basically, the approaches are the same, so go to newsapi.org and fill out the form for the new API key. Here I work with my previous one, so I log in!
After login, you will get a generated API key from the dashboard.
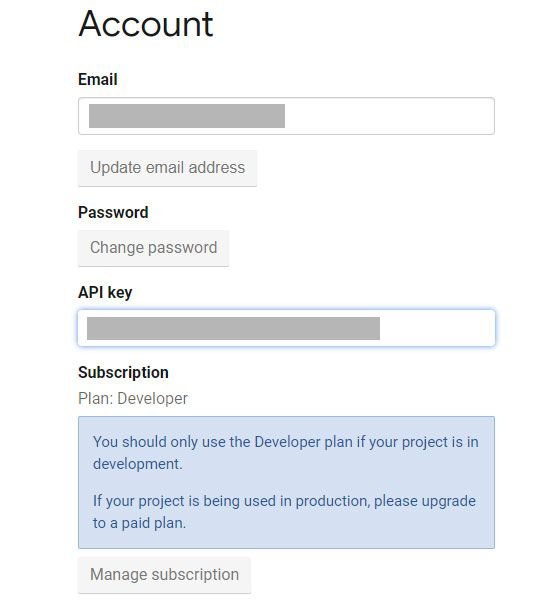
Don’t hesitate! feel free use any API key; just follow the rules and regulation provided by API
Axios
Axios is a lightweight, lightning-fast promised-based HTTP client based on HTTP service, similar to Native Fetch API.
CDN
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
NPM
npm install axios
YARN
yarn add axios
Import
axios.get('https://examples.com/example.json') .then((response) => { // handle success console.log(response); }) .catch((error) => { // handle error console.log(error); })
Fetch API
Fetch API provides an interface for fetching data. It will look familiar for someone who has previous experience of working on XMLHttpRequest (XHR). But now its more powerful and flexible features are included. Nowadays all most all modern browser has support on, Fetch API.
fetch("https://examples.com/example.json") .then(response => { if (!response.ok) { throw Error(response.statusText) } return response.json() }) .then(data => { console.log(data) }) .catch(error => console.error(error))
Fetch API in React
We have to need to make sure is to have create react app install. So the way to do this is to npm install -g for global create-react-app.
And I have previously installed on my system.
Quick Start
npx create-react-app project-name cd project-name npm start
Now we can run npm start or yarn start to view my application on localhost:3000. Here is my folder structure for the project.
Folder Structure
project-name/ README.md node_modules/ package.json public/ index.html favicon.ico src/ components/ NewsHeadlines/ Headlines.js SingleHeadlines.js App.css App.js App.test.js index.css index.js logo.svg
We create a new folder called /components and inside another nested folder for Headlines.js and SingleHeadines.js called /NewsHedlines, So make sure everything working fine as we add and changed App.js.
I added a Bootstrap 4.5.2 CDN for CSS only in index .html for some component styling, and remove all unnecessary comments to keep it simple!
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"/> <link rel="icon" href="%PUBLIC_URL%/favicon.ico"/> <meta name="viewport" content="width=device-width, initial-scale=1"/> <meta name="theme-color" content="#000000"/> <meta name="description" content="Web site created using create-react-app" /> <link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png"/> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <link rel="manifest" href="%PUBLIC_URL%/manifest.json"/> <title>React working with Axios and Fetch API</title> </head> <body> <noscript>You need to enable JavaScript to run this app.</noscript> <div id="root"></div> </body> </html>
Let’s start and create the first component Headline.js in /components/NewsHeadlines/ directory. First of all, we have to import react and call other components created just after the Headline component. Basically, in Headline components, we function for iterate and render data from our API and leverage the JavaScript map function.
And we know, when we’re iterating through components, we need a unique key to pass through each, and this one will be the URL from the item object that we’ll get here.
Now let’s export default Headlines!
Headline.js
import React, {Component} from "react"; import SingleHeadline from "./SingleHeadlines"; class Headlines extends Component { constructor(props) { super(props); this.state = { news: [] }; } componentDidMount() { const apiUrl = "https://newsapi.org/v2/top-headlines?sources=bbc-news&apiKey=63776d05d7374eea9f0e441a573b30a8"; fetch(apiUrl) .then((response) => { return response.json(); }) .then((data) => { this.setState({ news: data.articles }); }) .catch((error) => console.log(error)); } renderItems() { return this.state.news.map((item) => ( <SingleHeadline key={item.url} item={item}/> )); } render() { return <div className="row">{this.renderItems()}</div>; } } export default Headlines;
App.js
import React from "react"; import './App.css'; import Headlines from './components/NewsHeadlines/Headlines' export default function App() { return ( <div className="container-fluid my-5"> <h3 className="text-center mb-5"> React Working with Fetch API </h3> <Headlines/> </div> ); }
We create a stateless component for passing prop and returning some elements here. Basically, we iterate through the title. Now we haven’t any data, and it will not be going to working until passing some data through it. And therefore, the map function will not be working because items are undefined! Finally, we have to export default SingleHeadlines !
SingleHeadlines.js
import React from "react"; const SingleHeadline = ({item}) => ( <a className="col-md-3 mb-3" href={item.url}> <div className="card"> <img className="img-fluid" src={item.urlToImage} alt=""/> <div className="card-body"> <p className="card-title">{item.title}</p> </div> </div> </a> ); export default SingleHeadline;
Now I open pre-installed Postman for handling API requests from News API. From get request, we populate data from Postman like this screenshot and example also mentioned earlier this tutorial.
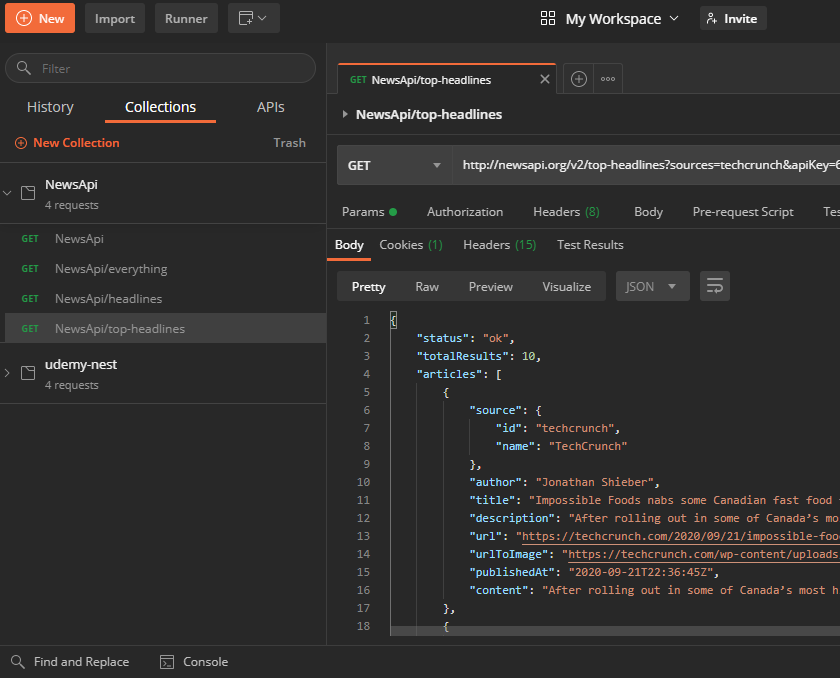
The first thing we’re going to create a state inside that component. To create a state, you must be familiar with this if you’ve made a couple of applications with React, so, constructor and we’re initializing the constructor with props, like so. And then we’re going to initialize our state, so this.state. And initialize it with news and create an array, like so.
Okay, so we’re good to go here. And then we’re going to use one of the lifecycle methods called componentDidMount, like so. What we’ll do inside of that function, and as you remember, this got a function that runs after the component has been rendered, and we want to do that to make sure that we can leverage the SingleHeadlines here, like so. So, we’ll create a variable called apiUrl, and we’ll paste the address that we just copied from News API.
Okay, excellent. So we got our URL created, now, what do we do with it? So, this is where the fetch function from ES6 is going to come into work, and this is where we’re going to be able to use even promises actually to fetch, and if there’s a response, then do something with this. If there’s no response, then catch the error, and so on. So, fetch, the fetch method actually takes a URL here, so you could copy this URL and put it here, but I created a variable for that purpose, so I’m going to pass that URL here.
And again, we have access to this from the data we get from Postman, so the URL is here. So this one would be an item.url. And then I always want to maintain a UI UX friendly thing where you want to keep the relationship in-between your user and where you bring them and where you brought them from. So basically, we always want to open whatever link to another tab or page.
Axios in React
A little bit update has been made for Headline.js in terms of Axios
import React, {Component} from "react"; import SingleHeadline from "./SingleHeadlines"; import axios from 'axios'; class Headlines extends Component { constructor(props) { super(props); this.state = { news: [] }; } componentDidMount() { const apiUrl = "https://newsapi.org/v2/top-headlines?sources=bbc-news&apiKey=63776d05d7374eea9f0e441a573b30a8"; axios.get(apiUrl) .then((response) => { this.setState({ news: response.data.articles }) }) .catch((error) => console.log(error)) } renderItems() { return this.state.news.map((item) => ( <SingleHeadline key={item.url} item={item}/> )); } render() { return <div className="row">{this.renderItems()}</div>; } } export default Headlines;
I install Axios using npm install –save axios command and import import axios from ‘axios’ on top of the file.
Here is the little bit update has been made for Axios
axios.get(apiUrl) .then((response) => { this.setState({ news: response.data.articles }) }) .catch((error) => console.log(error))
And the final file will look like this.
Headline.js
import React, {Component} from "react"; import SingleHeadline from "./SingleHeadlines"; import axios from 'axios'; class Headlines extends Component { constructor(props) { super(props); this.state = { news: [] }; } componentDidMount() { const apiUrl = "https://newsapi.org/v2/top-headlines?sources=bbc-news&apiKey=63776d05d7374eea9f0e441a573b30a8"; axios.get(apiUrl) .then((response) => { this.setState({ news: response.data.articles }) }) .catch((error) => console.log(error)) } renderItems() { return this.state.news.map((item) => ( <SingleHeadline key={item.url} item={item}/> )); } render() { return <div className="row">{this.renderItems()}</div>; } } export default Headlines;
Result
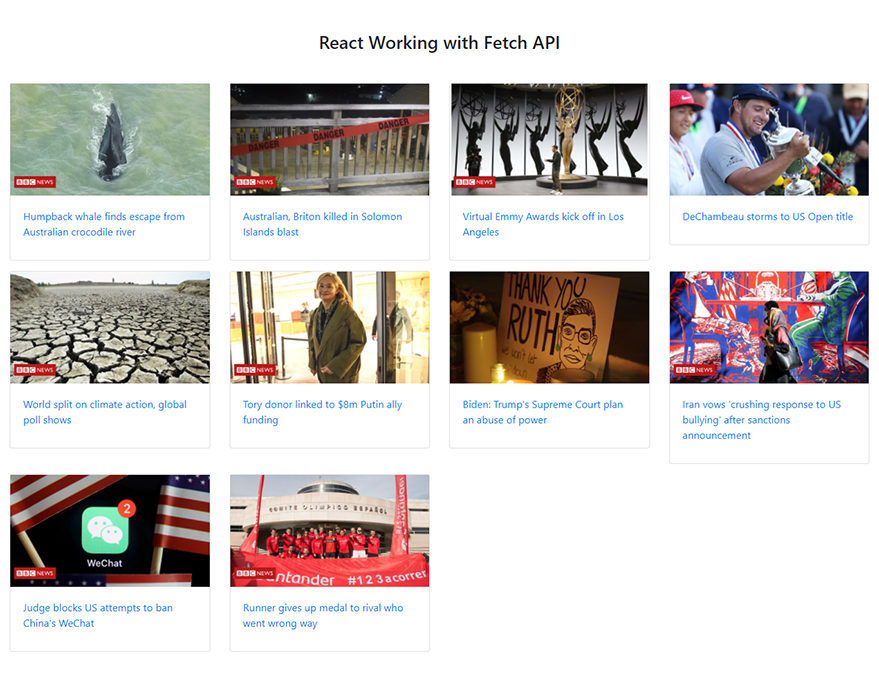
Conclusion
This tutorial was a journey of building a news portal, leveraging external APIs in React. With this knowledge, you should undoubtedly and quickly connect to all kinds of APIs with React and use the Axios and fetch methods we’ve explored. You could drive yourself and continue exercising by adding more APIs to build on your information gateway. Some APIs have more specifications, such as connecting with auth0, use unique authentication methods first, et cetera. For example, learning more about JWT, JSON Web Tokens will help you further when using these APIs with more security features.
Thanks very much for reading this article.
Resources and Tools
React Developer Tools
Project Source Code (Github)
Postman (The Collaboration Platform for API Development)
Axios
Fetch