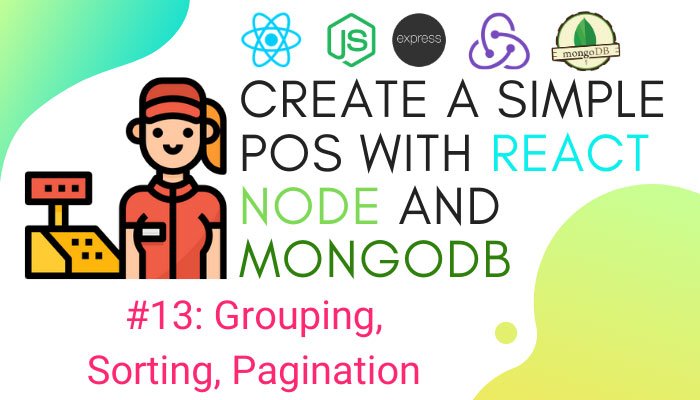
Defenition: POS – “Point of Sale”. At the point of sale, the merchant calculates the amount owed by the customer, indicates that amount, may prepare an invoice for the customer (which may be a cash register printout), and indicates the options for the customer to make payment.
In the previous chapter, we integrated react-table to our codebase and replaced the old table. We created a headless Table component so that we can import it to other components and use it easily. In this chapter, we are going to continue from where we left off from the previous chapter. Here, we are going to integrate grouping and sorting features to our react table along with pagination. Before we get started on integrating the respective features to the react-native, we need to work on moving the Table component and add it to the POS machine section. After adding it to the POS machine section, we are going to start with the Sorting feature first.
So, let us get started!
Sorting
In order to add a sorting filter to our react table, we are going to use useSortBy
hook. This will handle the sorting task. Hence, we need to import it from the react-table package as directed in the code snippet below:
import { useTable, useFilters, useSortBy } from "react-table";
Then, we need to add it to our main function as displayed in the code snippet below:
export default function Table({ columns, data }) { const [filterInput, setFilterInput] = useState(""); const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow, setFilter, } = useTable( { columns, data, }, useFilters, useSortBy );
Next, we need to attach the sort functionality to the table as directed in the code snippet below:
<th {...column.getHeaderProps(column.getSortByToggleProps())}> {column.render("Header")} <span> {column.isSorted ? column.isSortedDesc ? ' 🔽' : ' 🔼' : ''} </span> </th>
Hence, we will get the result as displayed in the demo below:
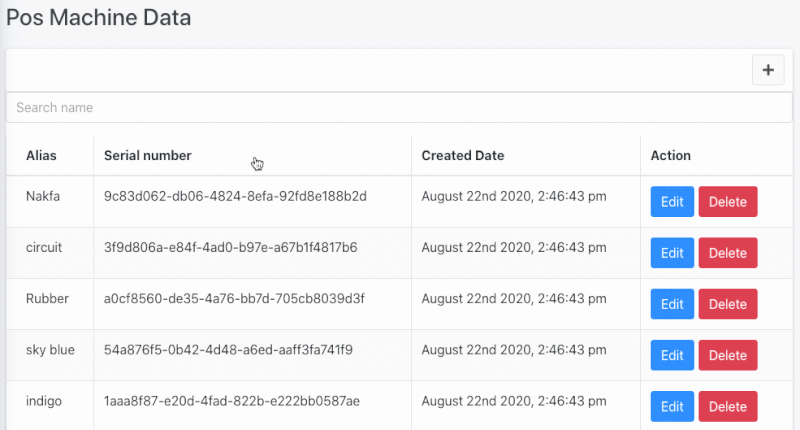
Now, we are able to sort the table data based on table headers.
Grouping
For the Grouping feature, we need two hooks. They are useGroupBy
and useExpanded
which we need to import from the react-table as shown below:
import { useTable, useFilters, useSortBy, useGroupBy, useExpanded} from "react-table";
Next, we need to attach them to the main method as highlighted in the code snippet below:
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow, setFilter, } = useTable( { columns, data, }, useFilters, useGroupBy, useSortBy, useExpanded, );
Now in order to use it in our table, we need to add it to the column header as directed in the code snippet below:
<th {...column.getHeaderProps(column.getSortByToggleProps())}> {column.canGroupBy ? ( // If the column can be grouped, let's add a toggle <span {...column.getGroupByToggleProps()}> {column.isGrouped ? '🛑 ' : '👊 '} </span> ) : null}
Hence, we will get the result as displayed in the following demo:
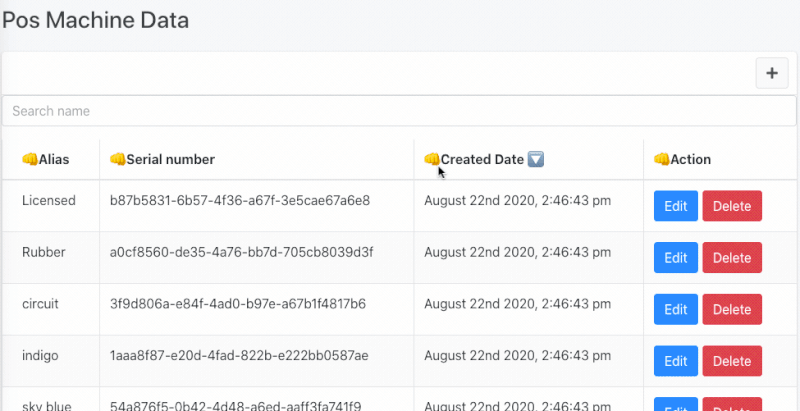
Here, we can see the count number on each column entry after grouping.
Pagination
Integrating pagination into our table requires a lot of work. First, we need to seed some data to our database. But, first, we need to install the faker plugin. For installation, we need to run the following command in our project terminal:
npm i faker
Now in api_pos_machine.js, we import the faker plugin as shown in the code snippet below:
var faker = require('faker');
Next, we need to work on the function code which is meant to add a new row to the table. In that function, we need to add the code from the code snippet below in order to generate the seed data:
let pos = []; for (let i = 0; i < 100; i += 1) { let alias = faker.random.word(); let serial_number = faker.random.uuid(); let newPos = { alias, serial_number, }; pos.push(newPos); // visual feedback always feels nice! console.log(newPos); } let doc = await POS_Machine.create(pos);
Here, we are iterating with limit 100 and adding a random word
and uuid
to the newPos
object variable. The newPost
is then added to the pos
array.
After seeding the data, we are going to see tons of row on the table as displayed in the screenshot demo below:
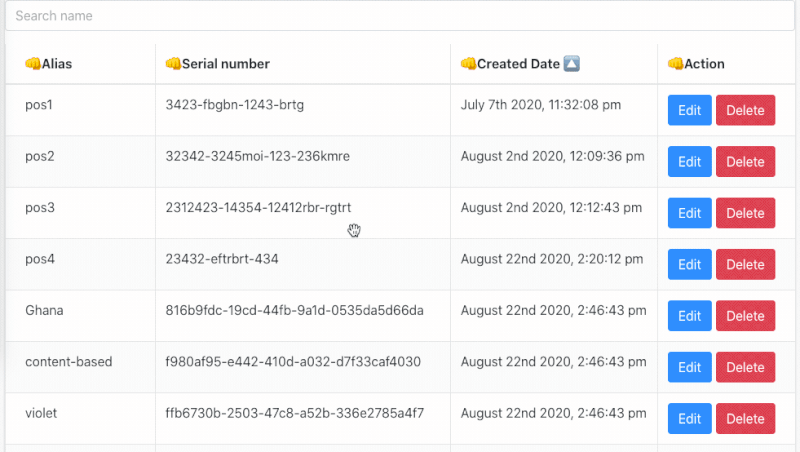
Now, we can start implementing pagination on our Table component.
First, we need to import usePagination
hook from the react-table package as directed in the code snippet below:
import { useTable, useFilters, useSortBy, useGroupBy, useExpanded, usePagination } from "react-table";
Then, we need to add all pagination functions to our main function as highlighted in the code snippet below:
export default function Table({ columns, data }) { const [filterInput, setFilterInput] = useState(""); const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow, setFilter, page, // Instead of using 'rows', we'll use page, // which has only the rows for the active page // The rest of these things are super handy, too ;) canPreviousPage, canNextPage, pageOptions, pageCount, gotoPage, nextPage, previousPage, setPageSize, state: { pageIndex, pageSize }, } = useTable( { columns, data, initialState: { pageIndex: 2 }, }, useFilters, useGroupBy, useSortBy, useExpanded, usePagination );
Now inside the table body, we need to change row
map to page
map as highlighted in the code snippet below:
<tbody {...getTableBodyProps()}> {page.map((row, i) => { prepareRow(row); return (
Next, we need to add pagination control at the bottom of the table view. We can add plain pagination control using HTML only. But fortunately, we have a Bootstrap CSS framework providing us with a modern and intuitive style for pagination control. This will save us tons of time on design. Here, we have three-part of pagination control. First for navigating between screens which are forward and backward navigation. This can be implemented using code from the following code snippet:
<form className="inline"> <div className="form-row"> <div className="form-group input-group col-md-2"> <ul className="pagination"> <li class={!canPreviousPage ? "page-item disabled" : "page-item "} > <a className="page-link" onClick={() => gotoPage(0)} >{'<<'}</a> </li> <li class={!canPreviousPage ? "page-item disabled" : "page-item "}> <a className="page-link" onClick={() => previousPage()} >{'<'}</a> </li> <li class={!canNextPage ? "page-item disabled" : "page-item "}> <a className="page-link" onClick={() => nextPage()} >{'>'}</a> </li> <li class={!canNextPage ? "page-item disabled" : "page-item "}> <a className="page-link" onClick={() => gotoPage(pageCount - 1)} >{'>>'}</a> </li> </ul> </div>
Hence, we will get the result as displayed in the following demo:
We can notice that the page changes along with page number as we click on forward and backward buttons. Secondly, we need to add the pagination control for forms to select the page that we need to navigate to. This can be implemented using the code from the following code snippet:
<div className="form-group input-group col-md-2"> <input className="form-control" type="number" defaultValue={pageIndex + 1} onChange={e => { const page = e.target.value ? Number(e.target.value) - 1 : 0 gotoPage(page) }} /> </div>
Hence, we will get the result as shown in the demo below:
Here, we can see that the page changes as we change the page number. Lastly, we need to add the pagination control to the page size. With this, we will be able to choose the number of rows that we need to see on the table. In order to implement this, we can use the code from the following code snippet:
<div className="form-group input-group col-md-2"> <select className="custom-select" value={pageSize} onChange={e => { setPageSize(Number(e.target.value)) }} > {[10, 20, 30, 40, 50].map(pageSize => ( <option key={pageSize} value={pageSize}> Show {pageSize} </option> ))} </select> </div> <span> Page{' '} <strong> {pageIndex + 1} of {pageOptions.length} </strong>{' '} | Go to page:{' '} </span> </div> </form>
Hence, we will get the result as displayed in the following demo.
Now, we can choose the number of rows that we want displayed on the table. Finally, we have successfully integrated the sorting, grouping, and pagination control features into our Table component.
Conclusion
We made our table view more intuitive and power-packed in this tutorial. We got the detailed stepwise guidance on integrating the sorting, grouping, and pagination control to our react table. We have the use of hooks along with the faker plugin to integrate the sorting and grouping. For pagination, we used bootstrap for styling purposes and integrated pagination control three-ways. These features in our table will make it more dynamic and full-fledged.
The coding implementations used in this tutorial chapter are available on Github for both frontend and backend.
See you in the next chapter! Good day folks!