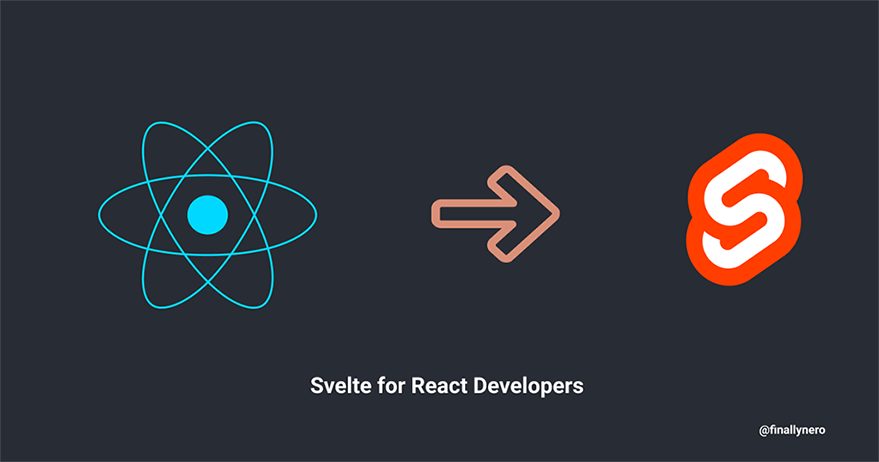
TL;DR: This article introduces Svelte to React developers. We will be explaining how different concepts in React like state, props, lifecycle methods are implemented in svelte. This will serve as a guide to get up and running with Svelte from a React developer point of view.
Introduction
Svelte is yet *another* SPA javascript framework in the ever-increasing list of Javascript frameworks. Oops sorry, ignore the canned introduction for javascript frameworks. Svelte is not a framework but a compiler. Svelte compiles the component code for your SPA at build time to highly-optimized vanilla Javascript and this is what is run on your browser.
Unlike React that ships with a virtual DOM that has to be calculated and reconciled every time the application’s state changes in the browser’s runtime. Svelte at compile-time keeps track of state that might change the DOM and whenever a piece of state changes it “surgically” updates the DOM using DOM API like createElement
& setAttribute
.
The lack of a virtual DOM is one of the more significant features of Svelte, this means svelte ships less code to the client. Also Svelte is a compiler so unlike React it doesn’t ship framework-specific API like this.setState
or useState
that has to be parsed and executed on the browser’s runtime. Svelte code shipped to the client is pure vanilla javascript.
Svelte’s small bundle size, performance, and awesome developer experience are some of the reasons why it stands out from the crowd of javascript frameworks released every second.
In this article, we’ll look at how common features in React like state, props, components e.t.c are implemented in svelte.
Components
Components in svelte are written in a .svelte
file. In this .svelte
file, you can write your HTML markup and it will be rendered unlike React where you have to create a function to return this markup.
Example:
//Hello.svelte <main> <h1>Hello World, Welcome to Svelte</h1> </main>
Svelte uses its templating language built on HTML to create the component user interface. While react uses JSX which is a javascript object that compiles to DOM nodes in the browser.
export default function App() { return ( <main> <h1>Hello World, Welcome to Svelte</h1> </main> ); }
What is a component without javascript(data/state) and styling?
Svelte give us a script tag where we will be writing javascript code/logic and a style tag where we write our CSS code. This is how a svelte component structure should look:
<script> // javascript lives here </script> <style> /* css/styling lives here */ </style> <!-- html lives everywhere else -->
The style block is where styles for this component are added and these styles are scoped to this component so don’t have to worry about clashing style classes in multiple components.
Component State
Component state is one of the reasons we use all these javascript frontend frameworks. We want to build interactive user interfaces that react to changes in data/state.
Creating a component state variable in Svelte is intuitive when compared to react. Let’s say we want to create a variable called `count` that would keep track of the number of times we click a button.
<script> let count = 0; function handleClick() { count += 1; } </script> <button on:click={handleClick}> Clicked {count} times </button> //Example from svelte documentation
Unlike React where we have to understand the useState
API in order to create a state variable, in Svelte we declare state variable the same way we do in vanilla Javascript.
Updating the state is even easier. We just assign the state to a new value. Then we added a click event on:click
which calls handleClick
function that updates the count by reassigning the count variable. The React equivalent would look something like this:
export default function Button() { const [count, setCount] = React.useState(0) const handleClick =()=>{ setCount((previousCount) => previousCount + 1) } return ( <button onClick={handleClick}>Clicked {count} times</button> ); }
No much difference in terms of lines of code but need an understanding of the useState
API in order to declare and update state in React.
Props
Props(Properties) is another commonly used feature in component-based frontend frameworks. Props allow you to pass data from a parent component to its children.
In React passing props looks something like this:
function Welcome(props) { return <h1>Hello, {props.name}</h1>; } function App() { return ( <div> <Welcome name="Nero" /> <Welcome name="Jack" /> <Welcome name="Bruno" /> </div> ); }; // Example from react documentation
Props passed to a component in React can be accessed in the child component from the props
object every component in React. In Svelte though the syntax seems a lot less intuitive.
// App.svelte <script> import Welcome from "./Welcome.svelte"; </script> <Welcome name="Nero" /> <Welcome name="Jack" /> <Welcome name="Bruno" />
// welcome.svelte <script> export let name; </script> <h1>Hello, {name}</h1>
When you pass a prop to a child component, you have to explicitly “accept” the props by using the export declaration. As seen in the example above, export
keyword informs the Welcome
component that name
is a prop
and not a state
value.
I know this looks awkward because export
in Javascript is usually used to export modules. However, In Svelte export
was modified to be used to declare props for a component.
Component Lifecycle methods
Components in component-based frameworks like React, Vue, Svelte e.t.c usually have a lifecycle in which they are created(mounted), updated, and destroyed (unmounted). This is similar to the cycle of life in animals i.e birth, growth, and death.
Svelte and React give us functions/callbacks that we can run at these different cycles in a component lifecycle.
The most popular lifecycle method for React developers would be the componentDidMount
, this is run immediately after the component is mounted on the DOM.
Most often than not this is where you initiate API calls to fetch data that is needed to initially render a component. The Svelte version of componentDidMount
is called onMount
.
Below is a table of the lifecycle methods in React and the Svelte version of them:
Svelte Lifecycle method | React Lifecycle method | Function/use case |
---|---|---|
OnMount() | componentDidMount() | This is called as soon as the component is mounted on the DOM |
OnDestroy() | componentWillUnmount() | This lifecycle hook is called just before the component unmounts and is destroyed. |
beforeUpdate() | – | This lifecycle hook is called before the DOM has been updated by state |
afterUpdate() | componentDidUpdate() | This lifecycle hook is called after the DOM has been updated by state |
– | shouldComponentUpdate() | It can be called if you need to tell React not to re-render for a certain state or prop change. Svelte’s architecture does not create a need for this kind of hook because it re-renders only the piece of the DOM that relies on the state that was updated. |
These are some of the lifecycle methods that we might need to use in development. You can check out the Svelte documentation for other lifecycle methods like tick.
Conditional Rendering
React developers handle conditional rendering using native javascript conditional statements like if/else, ternary operators, and switch statements. This is because JSX compiles to regular javascript function calls and evaluates to javascript objects so it is possible to use JSX inside regular javascript conditionals.
function Greeting({isLoggedIn}) { if (isLoggedIn) { return (<p>Welcome back</p>) }else{ return (<p>please sign in</p>) } }
However Svelte uses a template syntax to create the user interface. This template gives us a special syntax for conditionally rendering user interface components. Below is the Svelte version conditional rendering.
<script> export let isLoggedIn </script> <style> </style> {#if isLoggedIn} <p>Welcome back</p> {:else} <p>please sign in</p> {/if}
With Svelte we are stuck with this if/else
blocks as the only option for conditional rendering. This might take time to get familiar for React developers because we have a lot of options for conditional rendering, if/else blocks, ternary operator, switch
cases, and the dreaded short-circuit AND operator(&&).
Looping
Similar to how conditional are handled in Svelte. Iterating/looping over a list of data requires us to use a special each block syntax.
<script> let items = [{id: 1, name: 'nero'},{id:2, name: 'you'}] </script> {#each items as item} <p>{item.name}</p> {/each}
However, in React we have the freedom of using all the iterator expressions in javascript(map, for-loop, foreach e.t.c)
Data binding/ Controlled Inputs
According to Wikipedia Data-binding is a technique that binds data sources from the provider and consumer together and synchronizes them. Usually, HTML elements like input, textarea, and select maintain their own state and update the user interface based on what is entered. In some situations, we want React/Svelte to have access to this state and to be able to control the state of this element.
In some situations you want your component to be able to control the state of an input field. This is called a controlled input. Let’s take a look at how controlled inputs are implemented in React and Svelte.
React:
const SignupForm = () => { const [username, setUsername] = useState(' '); const handleChange = event => setUsername(event.target.value); return ( <label> username: <input type="text" value={username} onChange={handleChange} /> </label> ); };
A controlled input takes a value prop and an OnChange callback that changes the value in the state.
Svelte:
// SignupForm.svelte <script> let username = ""; function setUsername(event) { username = event.target.value; } </script> <label> username: <input type="text" value={username} on:input={setUsername} /> </label>
Above is the Svelte version of the controlled input. However, Svelte gives us a better way to implement two-way data binding. Svelte has a bind:value directive that directive binds the input to the component state without the need for an on:input callback to be defined. The above can be succinctly rewritten like :
// SignupForm.svelte <script> let username = ""; </script> <label> username: <input type="text" bind:value={username} /> </label>
This removes the need for a callback that updates the state because all the magic is done by bind:value
the directive.
Component Composition
Component composition is a pattern in component-based FE frameworks that helps us reuse code between components. Let us take a modal for example. A modal is a component that might be reused multiple times and more often than not it doesn’t know the structure of the content it is going to render ahead of time.
In React this can be achieved by the react children prop. A basic example demonstrating this :
function BaseModal(props) { return ( <div className=”modal”> {props.children} </div> ); }
Then other components create their own version of Modal using the base modal component.
function WelcomeModal() { return ( <BaseModal color="blue"> <h1 className=“modal-title"> This is the title </h1> <p className=“modal-content”> Here is the content </p> </BaseModal> ); }
Svelte doesn’t have a children
prop but it implements this pattern using an HTML tag called slots. Slots allow us to render HTML or other components inside a component.
A basic example of how component composition works in Svelte:
// BaseModal.svelte <script> //Javascript logic here </script> <style> /* css/styling lives here */ </style> <div className=”modal”> <slot></slot> </div>
We can then use the base modal in other components like this:
// WelcomeModal.svelte <script> import BaseModal from './BaseModal.svelte'; </script> <BaseModal> <h1 className=“modal-title"> This is the title </h1> <p className=“modal-content”> Here is the content </p> </BaseModal>
Other Svelte features
Svelte has some other interesting features that you might want to look at:
Conclusion
We have seen that a lot of features we use for development in react is also available in Svelte. I would give it to Svelte for making their APIs a little more developer friendly e.g declaring and updating component data. But I still need to get used to the template approach in terms of conditional rendering and looping. Svelte is an awesome frontend “framework”. Everyday people keep adopting it in their projects and the community keeps growing.
References
- What is Svelte’s underlying DOM manipulation strategy?
- Svelte tutorial
- Getting started with Svelte
- Component composition
“No much difference in terms of lines of code but need an understanding of the useState API in order to declare and update state in React.”
To really nail home the point about React requiring an understanding of the useState API, and in addition, the component lifecycle, I’d like to point out that there’s actually a bug in your example. Though it likely wouldn’t cause a problem in this simple case, it could result in a hard to pinpoint bug in a more complex case where the event is being fired more often / more quickly.
export default function Button() {
const [count, setCount] = React.useState(0)
const handleClick =()=>{
setCount(count+1)
}
return (
Clicked {count} times
);
}
The handleClick function sets the count state variable to `count+1`, where `count` is the value at the time of render. Let’s say `count=1` and you click the button twice before React has a chance to re-render the component. What will actually happen is `handleClick` will be called twice, and will run `setCount(count+1)` twice, but in both cases, `count` is equal to 1. So essentially we set count to 2 twice.
To solve this, we need to give a callback function to our setter.
export default function Button() {
const [count, setCount] = React.useState(0)
const handleClick = () => {
setCount((previousCount) => previousCount+1)
}
return (
Clicked {count} times
);
}
Instead of using the value that was defined on render, the argument passed into the callback function will be the true current value of the state variable. Meaning on the first call to handleClick, `previousCount` will be 1, and on the second call `previousCount` will be 2. This will result in the correct final value, even if the function gets called multiple times between renders.
Svelte’s syntax on the other hand does not require this knowledge of component lifecycle in order to accurately update state. You simply increment the variable, and Svelte does the rest.
Unfortunately these comments don’t support spaces at the beginning of a line, so the formatting of my previous comment was broken. To clarify, the only change is:
`setCount(count + 1)` should be `setCount((previousCount) => previousCount + 1)`
Thanks, Daniel for catching this.
I will update the article to capture this.
Thank you