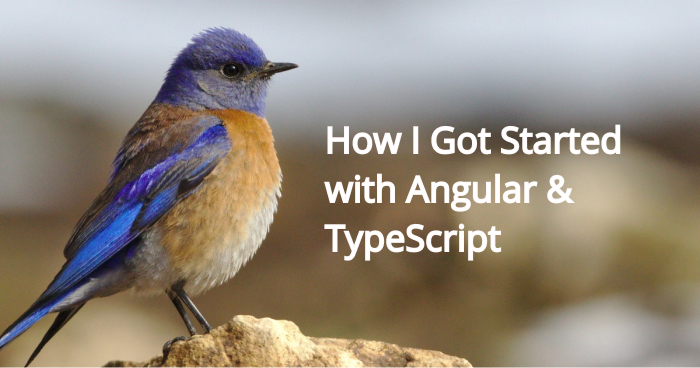
Learning Angular isn’t the easiest thing in the world. It takes a while to learn it. However, once I understood the basic architecture of Angular, creating apps with it became easier. In this article, I’ll show how I learned Angular and TypeScript without knowledge of either.
The Beginning
When I knew nothing, I started by writing practice apps with Angular. The fastest way to get started is using the Angular CLI. I created a basic app first by install the Angular CLI by running:
npm install -g @angular/cli
Then I created my first Angular app project by running:
ng new practice-app
In the Angular CLI, I chose to include routing. And choose to include CSS so reduce the amount of things I have to learn. Then we start our app by going to the practice-app folder and then run ng serve to start the development server that comes with Angular CLI.
Writing Some Basic Angular Code
Now that I have started our Angular CLI development server, I will write some code.
Showing Some Data on the Screen
I first practiced how to display some dynamic data on the screen from components. First, I went to app.component.ts . I see that it has the following content generated by the Angular CLI:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'practice-app'; }
I start by adding some fields to the AppComponent
class, which changed the code to:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { firstName = 'Jane'; lastName = 'Smith'; }
Then I went to app.component.html
, which has:
<!--The content below is only a placeholder and can be replaced.--> <div style="text-align:center"> <h1> Welcome to {{ title }}! </h1> <img width="300" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg=="> </div> <h2>Here are some links to help you start: </h2> <ul> <li> <h2><a target="_blank" rel="noopener" href="https://angular.io/tutorial">Tour of Heroes</a></h2> </li> <li> <h2><a target="_blank" rel="noopener" href="https://angular.io/cli">CLI Documentation</a></h2> </li> <li> <h2><a target="_blank" rel="noopener" href="https://blog.angular.io/">Angular blog</a></h2> </li> </ul> <router-outlet></router-outlet>
generated by Angular CLI. I changed that to:
<p>{{firstName}} {{lastName}}</p>
Then I see ‘Jane Smith’ on the screen. Next, I learn about adding the constructor method into the component class to initialize the component. I changed the code in app.component.ts
to:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { firstName: string; lastName: string; constructor() { this.firstName = 'Jane'; this.lastName = 'Smith'; } }
As we can see, whatever property of this can be shown in the corresponding HTML template if we put then between curly braces and without the
this
. So this.firstName in the component code becomes {{firstName}} in our HTML template. Likewise, we can display array items on the screen. The difference is that we have to use the *ngFor directive in our template to loop through the items and display them. To display a list of objects, first we have to create an interface to indicate the structure of the array entry that we want to display. We run ng g interface Person to create a Person interface in the person.ts file.
In person.ts
, we replace the existing code with:
export interface Person { name: string; }
Then we go back to app.component.ts
and change the file to:
import { Component } from '@angular/core'; import { Person } from './person'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { persons: Person[]; constructor() { this.persons = [ { name: 'Jane' }, { name: 'John' }, { name: 'Mary' }, ] } }
In the code above, we have the names array, which is of type Person[] . It’s an array of Person objects, where Person is the interface that we just created. The Person
interface specified that our Person
object has the
name
property and it’s of type string
.
Then we go to app.component.html
and change it to:
<p *ngFor='let p of persons'>{{p.name}}</p>
Then we get:
Jane John Mary
displayed on the screen. In the code above, we have the following expression in our *ngFor
directive:
let p of persons
p
has the entry from the this.persons array in app.component.html and {{p.name}} returns the name property of p so that we can display the name from there.
Conditional Display with NgIf
Next, I learned how to conditionally display items with the *ngIf directive. It takes an expression that returns a truthy or falsy value. I changed app.component.ts
to:
import { Component } from '@angular/core'; import { Person } from './person'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { displayName: boolean = false; }
Then I changed app.component.html
to:
<p *ngIf='displayName'>Jane</p>
Now I see nothing on the screen. I realized that this.displayName in app.component.ts set to false hides the name if we write *ngIf=’displayName’ . Then I set this.displayName to true in
app.component.ts
. Now I see the ‘Jane’ displayed without changing app.component.html . This means that the content is displayed if displayName
is true
.
Template Syntax
After looking at the Angular documentation, the curly braces is for interpolating data from our component file in our template. Anything between the double curly braces are interpolated. We can put any valid HTML in our template, along with variables, some expressions, and directive in our template code. I put expression straight into our template as follows:
app.component.html
:
{{1 + 1}}
Then I see 2 on the screen. It evaluated the 1 + 1 expression and returned the result. However, I after trying other things like assigning variables, operators, chaining expressions with ;
or ,
, increment and decrement operators, I see that they all give me errors. Bitwise operators also didn’t work for me. Also, I discovered that I can define reference variables in templates to access the element directly.
I changed app.component.html
to:
<label> {{input.name}}: <input #input name='foo'> </label>
I see that foo: is besides the input box. So now I know that #input sets the name of my input element to input . Then I can access its DOM properties directly, as I did with input.name to get the value of the name attribute that I’ve set.
Conclusion
I learned the most basic parts of Angular by trying various constructs myself. I created the app by running the Angular CLI. First I tried to display component field values on the screen with double curly braces in the template. Then I tried playing with component class fields and basic directives like *ngIf to conditionally display content and *ngFor to display an array of items. Finally, I set an HTML element to a variable and then accessed its attributes directly in the template.
Repos:
https://bitbucket.org/hauyeung/angular-basic-app-1/src/master/
https://bitbucket.org/hauyeung/angular-basic-app-2/src/master/
https://bitbucket.org/hauyeung/angular-basic-app-3/src/master/