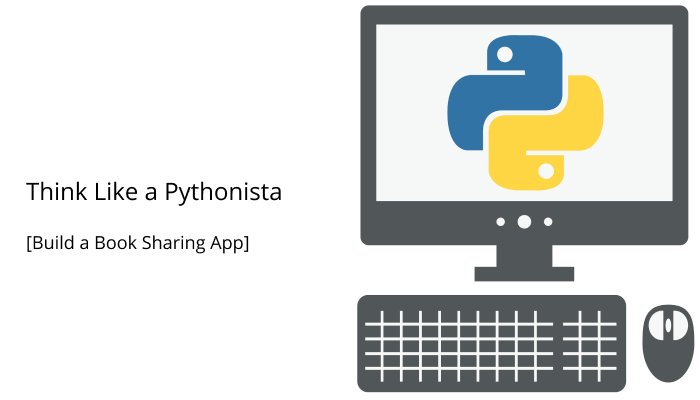
Python is at its hot seat — thanks to the boom of machine learning and artificial intelligence and startups wanting to implement their proof of concept quickly using a single language for the whole stack — the need for Python developers had skyrocketed in recent years.
As a result, a lot of people are moving towards learning Python. There is an insane amount of articles and tutorials online. I have been working with a lot of communities and taught Python to newbies who want to get into the industry. By working with people from a wide spectrum of college students to fresh graduates to people entering the industry after a break, the common pattern with all these tutorials is that they never get you beyond the syntactic side of python. While having these Python constructs at the back of your hand comes in handy while coding, that is not all it is to becoming a Pythonista.
Who is a Pythonista
A Pythonista is someone who uses Python programs to perform specific tasks. They know Python so well that when they look at the problem, thanks to Python’s English like constructs, they think through the solution completely in Python.
The tasks can be as simple as
- Searching Google
- Sending an email
- Scraping a website
- Reading a file
- Drawing charts
What is an Application?
An application is a software program written for end-users (people who are not into tech). In this case, imagine you as a software developer, and you are writing this app for a person who is a book freak.
There are different kinds of applications, mostly defined by the way in which the user interacts with the app.
- Command-line application
- Desktop application
- Mobile application
- Web application
- AR / VR application
In this blog, we are going to learn Python by building a book sharing application. We are going to build it as a command-line application the ones you can fire up on your terminal.
How to consume this blog?
Things are going to get heated now, treat this blog as a workshop where the instructor guides you step by step through the process. Hence, you are expected to hands-on. Boot up your laptop, open the blog on one side and try the exercises, code blocks and the other side. If you are stuck or find an error in the middle, check stackoverflow.com and Google the error messages — it will help you find your way. Most importantly, give it time, you might not be able to build this app at your first read, keep at it. Try it tomorrow, reach out to people for help, look into other tutorials. You will get there.
What makes an Application?
Whenever we talk about building an application, we broadly think about three things:
- Interaction
- Features
- Data
- Implementation
Interactions
- On starting the app, users are provided with a menu to choose whether they want to
list, add, edit or share
a book - User can choose “add” to add books that they have
- User can choose “list,” all books are listed
- On adding the books, they get stored
- When the user starts the app next time, the stored books should be preserved.
- User can “edit”/update the book details
- Once a book is added, user can “share” the book with their friends, for now, let’s just add a name under the label
SharedWith
Features
- List books
- Add books
- Update book
- Share
Data
Now, we take each item from the feature list and figure out what data we need to perform the operation on and how to design them. The idea of this section is to figure out.
- What to store?
- Where to store?
- How to store it?
List books
What to store
The minimum detail you need to list books is Book Name and Author
Where to store
We have different storage options like files, database, cloud, etc., Let’s stick with files to keep things simple.
How to store it
If you think about it, any file that lets us store data in a tabular format would come handy. That gives us two options CSV and Excel. CSV are text files with comma-separated values that look like this.
Title, Author Atomic Habits, James Clear Life is what you make it, Preethi Shenoy Power of Habits, Charles Duhigg
Add/Update Book
For adding or updating a book we don’t need any details beyond Title or Author
Share Book
When it comes to sharing a book,
- User might be willing to share a book only if they have already read it
- User needs to store details of a person who they shared the book with
So, for the book sharing app, we are storing Title, Author, IsRead, SharedWith
Setup
System Setup
We need a few pre-requisites before we start building the app:
- Of course, a laptop
- Install Python 3.7
python
command working in your terminal- Your favorite code editor, I use VSCode.
Project Setup
- Create a folder say
book-app
- Create a file with the name
app.py
- Create a file
books.csv
this is where we store our booklist
MindSet
- A lot of enthusiasm and curiosity
- A good chunk of time
- Good Wifi
- Maybe a shot of coffee
Implementation
Let’s split the features into smaller tasks and look at their Python implementation.
Listing the menu – On running app.py using python app.py
command we would show the user a bunch of options to choose from. We can do this using python print
statements
print("Menu ::") print("1. Add Book") print("2. Update Book") print("3. Share Book") print("4. List Book")
Choosing an option – Next, we need to let the user choose an option 1,2 or 3
. We can use python’sinput
command to get input from the user. Also, we need to store this option somewhere so that we can perform it later. We can store it in a python variable.
choice = int(input("Enter your option ::"))
Now on knowing what the choice is, we need to call respective Python function
.
if choice == 1:
add_book()
elif choice == 2:
update_book()
elif choice == 3:
share_book()
elif choice == 4:
list_books()
For each function call
we need to write the corresponding function definitions
Add Book
- For us to add a book we need to get its name and author from the user
- Next, we need to add this book details to
book.csv
file for that we need to understand
Functions should be defined before calling, make sure to add next chunks of code before the if
statements
def add_book(): # 4.1 book_name = input("Enter book name") author = input("Enter Author name") # 4.2 import csv # Opens the file in writing mode with open('books.csv', mode='a') as f: writer = csv.DictWriter(f, fieldnames=[ "BookName", "Author", "Read", "SharedWith"]) writer.writerow({"BookName": book_name, "Author": author})
Update Book
- Get the name of the book to be updated from the user
- Get the details that need to be updated, for now, let’s just keep it to marking the book as read or not read
- Read the books from the file and find if the book already exists in the
- If it does, update the detail
def update_book(): # 5.1 book_name = input("Enter book name ::") # 5.2 is_book_read = input("Book Read (Y/N)?") if is_book_read == "Y": is_book_read = True elif is_book_read == "N": is_book_read = False # 5.3 import csv rows = [] # Opens the file in reading and writing mode with open('books.csv', mode='r') as f: rows = list(csv.DictReader(f)) # 5.4 for row in rows: if row["BookName"] == book_name: row["Read"] = is_book_read break with open('books.csv', mode='w') as f: csv_writer = csv.DictWriter( f, fieldnames=["BookName", "Author", "Read", "SharedWith"]) csv_writer.writerows(rows) print("Book Successfully Updated")
Share Book – It is exactly the same as the update_book
function, except you, update the field SharedWith
your friend’s name. At this point, I challenge you to copy-paste edit_book
and update the functionality
List Books – We have also seen parts of what list books need to do in previous functions
- Read the file and get all the books
- Loop through the books and print them
import csv def list_books(): # Opens the file in reading and writing mode with open('books.csv', mode='r') as f: rows = csv.DictReader(f) for row in rows: print("\n") print("Book Name :: " + row["BookName"]) print("Author :: " + row["Author"]) print("Read :: " + row["Read"]) print("SharedWith :: " + row["SharedWith"])
With that, we have completed implementing all our features. The final code looks at this.
import csv def list_books(): # Opens the file in reading and writing mode with open('books.csv', mode='r') as f: rows = csv.DictReader(f) for row in rows: print("\n") print("Book Name :: " + row["BookName"]) print("Author :: " + row["Author"]) print("Read :: " + row["Read"]) print("SharedWith :: " + row["SharedWith"]) def update_book(): # 5.1 book_name = input("Enter book name ::") # 5.2 is_book_read = input("Book Read (Y/N)?") if is_book_read == "Y": is_book_read = True elif is_book_read == "N": is_book_read = False # 5.3 rows = [] # Opens the file in reading and writing mode with open('books.csv', mode='r') as f: rows = list(csv.DictReader(f)) # 5.4 for row in rows: if row["BookName"] == book_name: row["Read"] = is_book_read break with open('books.csv', mode='w') as f: csv_writer = csv.DictWriter( f, fieldnames=["BookName", "Author", "Read", "SharedWith"]) csv_writer.writerows(rows) print("Book Successfully Updated") def add_book(): # 4.1 book_name = input("Enter book name") author = input("Enter Author name") # 4.2 import csv # Opens the file in writing mode with open('books.csv', mode='a') as f: writer = csv.DictWriter(f, fieldnames=[ "BookName", "Author", "Read", "SharedWith"]) writer.writerow({"BookName": book_name, "Author": author}) print("Book Successfully Added") print("Menu ::") print("1. Add Book") print("2. Update Book") print("3. Share Book") print("4. List Book") choice = int(input("Enter your option ::")) if choice == 1: add_book() elif choice == 2: update_book() elif choice == 3: share_book() elif choice == 4: list_books()
Here are a few things you can try next:
- Maintain the record of
BookStartDate
andBookEndDate
- For each book, you can create a new file to create notes and store the name of the file under
Notes
inbooks.csv
- Come up with your own crazy feature, figure out the data, interactions, and deep dive into the code.
If you have come this far into the blog, give yourself a pat on the back; if you have successfully implemented the code and could finish the exercises then you are already way ahead of most people trying to learn Python. Next up, you can use my How to build your 1st web application tutorial to build your first web app. If you found this tutorial helpful, give me a shoutout on Twitter.
P.S. Project’s GitHub repo: https://github.com/bhavaniravi/book-app-python-tutorial
I don’t really have anything to say about the post itself, but I noticed a minor bug in your code: The correct way to open files for csv reading/writing is to use newline=”, i.e. open(‘books.csv’, mode=’r’, newline=”). The reason for this is documented in the footnote https://docs.python.org/3/library/csv.html#id3