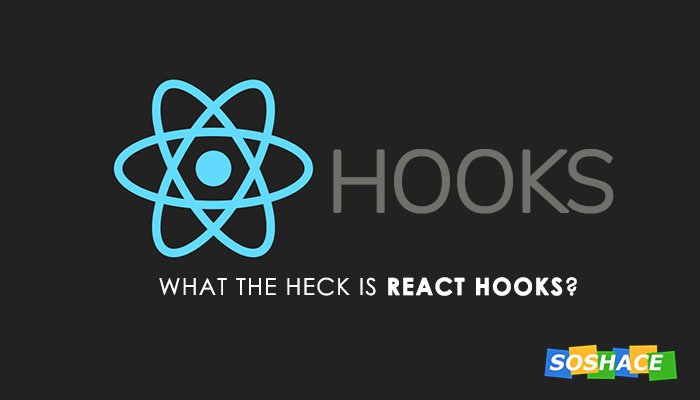
React Hooks allows functional components to hook into the react state and lifecycle methods. This was only possible in class components.
If you’ve worked on class components for a while, you’d be used to how cumbersome the structure would be because of state handling or lifecycle methods control. Although you may opt-in for separating different state concerns in different class components but that’s rarely done. One class component usually handles a lot of states and lifecycle methods at once.
With this awesome invention, most of those concerns can be handled directly from the functional (also with lesser structure) components. Isn’t this a miracle?
React 16.8.0 was the first version to support this feature.
Why Should I use this instead of Class Components?
More to the fact that the length of code structure is reduced,
- Complex components become easily understandable
- States are easily handled as every component can control its own state.
- Side effects are easily controlled.
React Hook APIs
React Hooks provides us with several APIs such as
- State Hook – useState
- Effect Hook – useEffect
- Context Hook – useContext
- Reducer Hook – useReducer
- and so many more. Find a list of all API references in the API documentation
We’d look at the first two APIs in this article because they are the most used.
State Hook
As you would remember, states were only handled in class components. For example, a drawer handler for holding nav links. Let’s say we already have a drawer component, we can control it like so;
import React from 'react'; import Drawer from './Drawer.js'; class MyComponent extends React.Component { state = { drawer: 'open' } openDrawer() { this.setState({ drawer: 'open' }) } closeDrawer() { this.setState({ drawer: 'close' }) } render() { return ( <React.Fragment> <button onClick={this.openDrawer}> Open </button> { this.state.drawer === 'open' ? ( <React.Fragment> <button onClick={this.closeDrawer}> Close </button> <Drawer /> </React.Fragment> ) : null } </React.Fragment> ) } }
Remember in react, when the state changes, the component is rendered again. When the button changes the state by calling the methods, the component is re-rendered.
As seen above, the class component is handling only one concern which is even rarely the case. There’s often more than one state to be handled. But the state hook makes it easier. Let’s implement the above with a functional component using the state hook – useState()
.
As beneficial as this hook is, you also need to understand the method of implementation. First, let’s see the contents of useState()
by logging it to the console.
import React, { useState } from 'react'; export default () => { console.log(useState(0)); return ( <h1>useState</h1> ) }
We get this array – [0, dispatchAction()]
We passed 0 as the argument, hence we get 0 as the first value of the array. This refers to the current state used for the first render. We also get a dispatchAction() used to update the state. This function can be called from an event handler. What it does is similar to this.setState({…}) in a class component.
With array destructuring, we could rename the contents of the array as we desire. Since we are trying to implement a drawer, we could have the following;
const [ drawerStatus, setDrawer ] = useState("close");
Remember that unlike object destructuring where which we can only destruct the keys, different names can be specified for array destructing.
For our handling drawer component, we would have the following;
import React, { useState } from 'react'; import Drawer from './Drawer.js' export default () => { const [ drawerStatus, toggleDrawer ] = useState("close"); return ( <React.Fragment> <button onClick={() => drawerStatus === 'open' ? toggleDrawer("close") : toggleDrawer("open")}> Toggle Drawer </button> { drawerStatus === "open" ? <Drawer /> : null } </React.Fragment> ) }
The above should be convincing. The code structure is small and everything concerning drawer is handled in this simple component. First, we pass a value of close meaning that the drawer is closed. Remember, it’s the first state when the component renders. We listen to the event on the button which calls an anonymous function. If the drawer status is open, clicking the button should change the drawer status to close. When the state changes, the component is re-rendered and whether to show the draw or not is determined.
More than 1 state hook
More than one state can be used in a component. To do this, different state names and function names would be used. For example;
const [ drawerStatus, toggleDrawer ] = useState("close"); const [ isOnline, changeStatus ] = useState(false);
Effect Hook
The effect hook allows us to perform side effects in functional components. Side effects refers to anything that affects something (usually other components) outside the scope of the function being executed. For example, network requests or manually setting the DOM. These cannot be done during first rendering but after.
Side effects are usually handled in three component lifecycle methods – componentDidMount()
, componentDidUpdate()
and componentWillUnmount()
. These three methods are unified and accessed with the Effect Hook. In other words, this hook performs the same purpose as these three methods.
The only way of adding side-effects was by using a class component. In the class component above (first example), we could have a lifecycle method – componentDidMount()
before the render method like so;
import React from 'react'; export default class MyComponent extends React.Component { // other codes componentDidMount() { setTimeout(() => this.setState({ drawer: 'open' }), 1000) } render() { return ( ... ) } }
What the above does is that after the component is rendered (mounted), the state of the drawer is changed. The delay of 1 second is added so that the change could be noticed.
Let’s see how the effect hook handles this. Still using the functional component above, we would have the following
import React, { useState, useEffect } from 'react'; import Drawer from './Drawer.js' export default () => { const [ drawerStatus, toggleDrawer ] = useState("close"); useEffect(() => { setTimeout(() => toggleDrawer("open"), 1000) }) return ( <React.Fragment> <button onClick={() => drawerStatus === 'open' ? toggleDrawer("close") : toggleDrawer("open")}> Toggle Drawer </button> { drawerStatus === "open" ? <Drawer /> : null } </React.Fragment> ) }
What the above does is that when the component is mounted, the drawerStatus
is changed to “open” after 1 second.
But our component has an issue. Even if the buttons change the state, the hook will change the status to “open” again. Remember that useEffect()
also does the work of componentDidUpdate()
which is run after every update. We’d look at a method for making sure it is only called after the component is mounted later in the article. For a consistent example, you can use the following instead the above
useEffect(() => { console.log(`The current drawer status is ${drawerStatus}`) })
Do not forget – This hook is run after every render (mount, updates or unmount) except further configured (which we would soon see).
Watching for changes in Effect Hook
If you remember with componentDidUpdate()
, you could have the following;
componentDidUpdate(prevProps, prevState) { if(prevState.id !== this.state.id) { // do something like fetching details about the // id from an api } }
We ensure that the id state is changed before performing any action on it. This is done for performance reasons so that you don’t end up doing the same thing over and over for an unchanged state. Effect hook also allows us to perform this comparison. To do so, pass an array as a second argument to the function which would contain a list of state variables to watch changes for. For our example, we’d have;
useEffect(() => ( console.log(`The current drawer status is %{drawerStatus}`) ), [drawerStatus])
This watches for any change in the drawerStatus
state.
You can also pass an empty array. This means that the hook will only be run on mount time – componentDidMount()
More than 1 effect hook
Just like the state hook, you can have more than one effect hook. For example;
useEffect(() => ( console.log(`The current drawer status is %{drawerStatus}`) ), [drawerStatus]) useEffect(() => ( console.log(`The component has mounted`) ), [])
As expected, the first hook is called on every render but the second is only called on the first render.
Rules of Hooks
Hooks have their rules which must be followed before they can be used. If not used properly, there’d be errors. In the console, you’ll see an error stating that the rules of hooks must be followed.
It has 2 rules;
- Only call hooks at the top level. Not inside if or loop statements. Don’t do this;
if(condition) { const [count, setCount] = useState(0); }
- Only call hooks from React function components, not normal functions or class components
Conclusion
There are many other hooks that give functional components powers. You can check out more APIs in the documentation.
If you aren’t already, I hope I’ve given you reasons why you should give this hook thing a try.
Thanks for reading : )
Hi, Dillion, thanks for an article! You forgot to mention custom hooks)
You’re welcome.
I intentionally left out other types of hooks to focus on useState and useEffect.
Oh, I see!
Function based components and hooks look like unmaintainable spaghetti code after a while and on larger projects class based comps are much more readable. Also using something like MobX or MST, there is not much State and lifecycle going on in those components – at least there shouldn’t. Otherwise you end up in a props drilling, lifecycle mess.
For smaller and less complex solutions, hooks and function components are fine though.