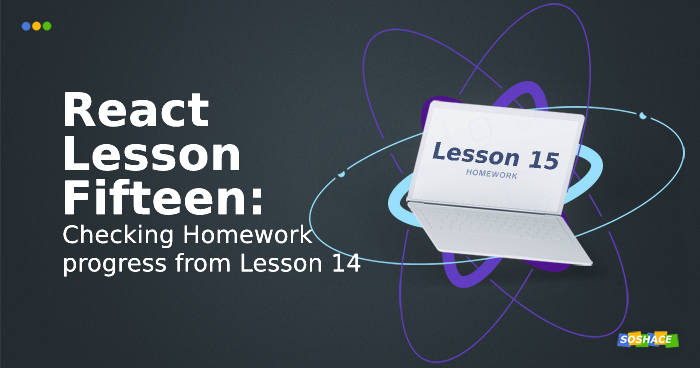
Hey everyone, this is the 15th and the last lesson of this React course and I hope you had fun learning along with me. Today, we are going to cover homework from Lesson 14 and add comment API to load comments. We will go through 4 simple steps:
- Create action types
- Add action to fetch comments from API
- Update reducer to receives these comments
- Update View to render the comments
Let’s start 🤩
Step 1: Create action types
As you may recall from Lesson 13, we need 3 types of action creators to handle 3 phases of request: start, fail, and success. Let’s create then:
// src/types.js export const FETCH_COMMENT_REQUEST = "fetch_comment_request"; export const FETCH_COMMENT_SUCCESS = "fetch_comment_success"; export const FETCH_COMMENT_FAILURE = "fetch_comment_failure"
Step 2: Add action creators to fetch comments from API
First, we will import the types we just created and define the action creators for the 3 phases of request. We have to dispatch one action creator when the request starts, one when the request fails, and finally one when the request is successful. Let’s add these:
import { ADD_COMMENT, FETCH_COMMENT_REQUEST, FETCH_COMMENT_SUCCESS, FETCH_COMMENT_FAILURE } from "../types"; // import types // to dispatch when the request starts const requestStart = () => { return { type: FETCH_COMMENT_REQUEST, }; }; // to dispatch when the request is successful const requestSuccess = (data) => { return { type: FETCH_COMMENT_SUCCESS, payload: data, }; }; // to dispatch when the request is failed const requestFailed = (error) => { return { type: FETCH_COMMENT_FAILURE, error: error, }; };
We are using redux-thunk to handle async requests just like we did with articles API. Now, we just have to add the thunk functions which will handle the asynchronous API call and dispatch the 3 actions we just created accordingly.
// add thunk to handle request export const fetchCommentRequest = (articleId) => { return function (dispatch) { dispatch(requestStart()); //dispatch when request starts return fetch(`http://localhost:3001/api/comment?article=${articleId}`) .then( response => response.json() ) .then(json => dispatch(requestSuccess(json)) //dispatch when request is successful ) .catch(error => dispatch(requestFailed(error)) //dispatch when request is failed ); } };
That’s it for the action creators. Please note, the comments are coming from http://localhost:3001/api/comment?article=${articleId}. This API endpoint receives articleId and returns all the relevant comments for that particular article. We will call this API when the user presses the “SHOW COMMENTS” button. Let’s move on to the 3rd step and update our reducer.
Step 3: Update reducer to receives these comments
We have changed the way how we used to get comments. Now, we are not getting all the comments from fixtures.js thus it is no longer needed. That means defaultComments will be an empty array and we will fetch only those comments which are requested by the user. Let’s jump to code now:
// replace normalizedComments with empty array [] const defaultComments = recordsFromArray(Comment, []);
The comments array can have all the comments of one article at a time. Hence, the INITIAL_STATE will be changed like this:
const INITIAL_STATE = { comments: defaultComments, isFetching: false, // add flag to know the status of request errors: [], // store error if API fails };
As we defined 3 action creators to handle the 3 phases of request. We will need 3 separate reducer cases to handle those action creators. Finally, let’s update the reducer to support the 3 action creators we wrote above:
export default (state = INITIAL_STATE, action) => { //set isFetching to true when the request starts case FETCH_COMMENT_REQUEST: return { ...state, isFetching: true }; //set error when the request fails case FETCH_COMMENT_FAILURE: return { ...state, isFetching: false, errors: state.errors.push(action.error) }; // update comments array with the data we got from API case FETCH_COMMENT_SUCCESS: return { ...state, isFetching: false, comments: recordsFromArray(Comment, action.payload) }; default: return state; }
Now, we just have to call the API with the requested articleId and get relevant comments.
Step 4: Update View to render the comments
Let’s understand what’s happening here. The user clicks on an Article title and sees the Article description along with the “Show Comment” button. Then “Show Comment” is clicked which will toggle the CommentList component to render. The CommentList.js component is responsible to list down all the comments.
We can use the componentDidMount method of the CommentList component to request the comments as soon as it is rendered and show the “loading…” text while the fetch is in progress.
We are going to dispatch the fetchCommentRequest action creator from this component and wire it with the “SHOW COMMENTS” button. Go to CommentList.js and import the action creator:
//import fetchCommentRequest import { addComment, fetchCommentRequest } from "../actions";
Now, call this action when the component mounts:
componentDidMount(){ this.props.fetchCommentRequest(this.props.article.id); }
Update render method to show “Loading…” text when API is in progress:
render() { // get comments and isFetching const { comments, isOpen, toggleOpen, article, addComment, isFetching } = this.props; //show loading text request is in progress if (isFetching) return <h3>loading...</h3>; // show this text if comments are not present if (!comments.length) return <h3>no comments yet</h3>; // list the comments const commentItems = comments.map(comment => { return ( <li key={comment.id}> <Comment comment={comment} /> </li> ); }); //...other part of code }
Update mapStateToProps method to get the comments and isFetching from INITIAL_STATE:
const mapStateToProps = (state, props) => { return { comments: state.comments.comments.valueSeq(), isFetching: state.comments.isFetching, }; };
And finally, connect mapStateToProps and the actions:
export default connect( mapStateToProps, { addComment, fetchCommentRequest } )(toggleOpen(CommentList));
The CommentList will look something like this, make sure you have not forgotten anything:
import { addComment, fetchCommentRequest } from "../actions"; class CommentList extends Component { componentDidMount(){ this.props.fetchCommentRequest(this.props.article.id); } render() { const { comments, isOpen, toggleOpen, article, addComment, isFetching } = this.props; if (isFetching) return <h3>loading...</h3>; if (!comments.length) return <h3>no comments yet</h3>; const commentItems = comments.map(comment => { return ( <li key={comment.id}> <Comment comment={comment} /> </li> ); }); const body = isOpen ? ( <div> <ul>{commentItems}</ul> <CommentForm articleId={article.id} addComment={addComment} /> </div> ) : null; const linkText = isOpen ? "close comments" : "show comments"; return ( <div> <button onClick={toggleOpen} ref="toggler"> {linkText} </button> {body} </div> ); } } const mapStateToProps = (state, props) => { return { comments: state.comments.comments.valueSeq(), isFetching: state.comments.isFetching, }; }; export default connect( mapStateToProps, { addComment, fetchCommentRequest } )(toggleOpen(CommentList));
We are showing a loading… text while the request is in progress. It’s a good practice to let the user know that we are fetching something. Once the request is complete, the isFetching flag will be false and comments will be shown to the user. We can also show an error if the request gets failed. At last, go to src/components/Article/index.js and update CommentList.js usage to support the new implementation:
//...above code <CSSTransition in={isOpen} timeout={500} classNames="article" unmountOnExit > <section> {text} <CommentList article={article} /> </section>
Here, we removed props comments in CommentList as it will be fetched directly when the component mounts for a given article.
Run the app and see if everything is working as expected.
You can update all the actions to support APIs as you already understand how async calls work with redux. The simple_api folder has all the APIs already created. You can use them to add comments, delete comments, add articles, delete articles, etc. The flow will be exactly similar to what we have just learned.
Thunk Middleware can dispatch as many actions as we want and it is very handy when it comes to handling async requests but it’s not the only way. Other popular methods include:
- redux-saga is another popular library which uses generator functions
- redux-observables dispatches observables which can then be observed for changes
- redux-promise to dispatch promises instead of functions
You can choose whatever you want based on your preference. Just make sure to be consistent and follow one method throughout the app. It will help you manage the state when the app grows bigger and becomes complex.
This is the end of this lesson as well as this course. I hope you had fun while building this app and learning the react concepts. We can’t wait to see you on soshace.com. Goodbye until we meet again 🙂.
You can find the complete code in this repo.