Sending Emails in Java Applications: A Comprehensive Guide to JavaMail and SMTP Configuration
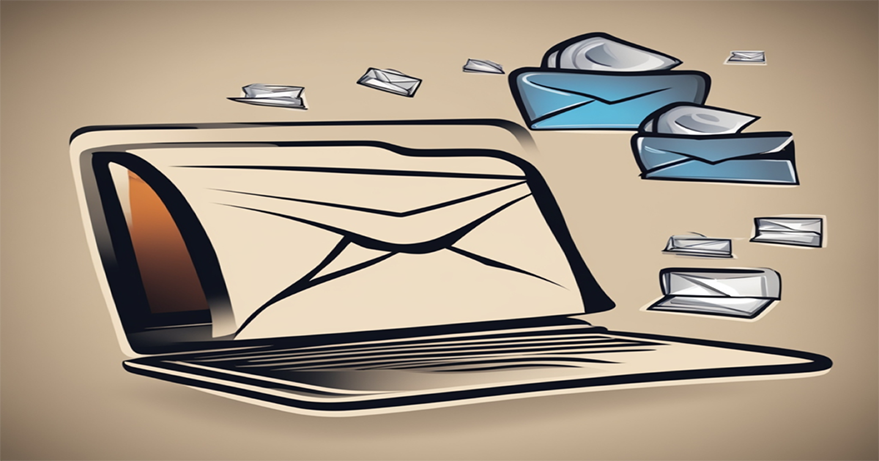
Sending Emails in Java Applications: A Comprehensive Guide to JavaMail and SMTP Configuration
Table of Contents
- Introduction
- Setting Up Your JavaMail Environment
- Understanding JavaMail Components
- Configuring SMTP for JavaMail
- Sending Simple Emails
- Advanced Email Features
- Handling Email Responses
- Best Practices for Email Sending in Java
- Troubleshooting Common Issues
- Conclusion
1. Introduction
1.1 Why Email Communication in Java Applications?
Email communication is a vital aspect of modern applications, facilitating various functionalities like user notifications, password resets, and transactional emails. Integrating email capabilities into Java applications enhances user experience and ensures effective communication between the application and its users.
1.2 JavaMail API Overview
The JavaMail API, part of the Java EE (Enterprise Edition) platform, simplifies the process of sending and receiving emails in Java applications. It provides a set of classes and interfaces for working with email protocols, making it a go-to solution for developers looking to implement robust email functionality.
2. Setting Up Your JavaMail Environment
2.1 Downloading and Including JavaMail Library
Before getting started, download the JavaMail library from the official Oracle website or your preferred repository. Once downloaded, include the JAR files in your Java project. For example, if you’re using Maven, add the following dependency to your pom.xml:
1 2 3 4 5 6 7 8 9 10 11 12 13 | <dependencies> <!-- Add JavaMail API dependency --> <dependency> <groupId>com.sun.mail</groupId> <artifactId>javax.mail</artifactId> <version>1.6.2</version> </dependency> <dependency> <groupId>com.sun.mail</groupId> <artifactId>jakarta.mail</artifactId> <version>1.6.7</version> </dependency> </dependencies> |
2.2 Configuring Your Java Project
Configure your Java project to use the JavaMail API. If you’re using an Integrated Development Environment (IDE) like Eclipse or IntelliJ, make sure to add the JavaMail library to your project’s build path.
3. Understanding JavaMail Components
3.1 Session
The Session class in JavaMail represents a mail session, which encapsulates the properties and settings for connecting to a mail server. To create a Session instance, you need to use the Session.getInstance() method:
1 2 3 4 5 6 7 8 9 10 11 12 13 | import javax.mail.Session; import java.util.Properties; public class EmailSender { public static void main(String[] args) { Properties properties = new Properties(); properties.put("mail.smtp.host", "your-smtp-host.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); Session session = Session.getInstance(properties); } } |
3.2 Message
The Message class represents an email message. To create a simple text message, use the MimeMessage class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | import javax.mail.Message; import javax.mail.MessagingException; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; public class EmailSender { public static void main(String[] args) throws MessagingException { // Assuming 'session' is a configured Session instance Message message = new MimeMessage(session); message.setSubject("Hello, JavaMail!"); message.setText("This is a test email from JavaMail."); // Now 'message' is ready to be sent } } |
3.3 Transport
The Transport class handles the actual delivery of email messages. To send a message, use the Transport.send() method:
1 2 3 4 5 6 7 8 | import javax.mail.Transport; public class EmailSender { public static void main(String[] args) throws Exception { // Assuming 'session' and 'message' are configured instances Transport.send(message); } } |
3.4 Store
The Store class in JavaMail is a fundamental component that facilitates the retrieval of email messages from a mail server. While our focus in this guide has primarily been on sending emails, understanding how to retrieve and manage incoming messages is equally important for comprehensive email functionality in Java applications.
IMAP: IMAP is a widely used protocol for retrieving emails from a server. It allows users to view and manipulate messages without downloading them to the local machine. IMAP maintains the messages on the server, providing a synchronized view across multiple devices.
POP3: POP3, on the other hand, is another protocol for email retrieval. Unlike IMAP, POP3 downloads emails to the local machine, removing them from the server. This makes it suitable for scenarios where emails are primarily accessed from a single device.
3.4.1 Connecting to a Mail Server
To use the Store class, you first need to obtain a Session object (similar to what we discussed in previous sections). The Session object encapsulates the properties required for establishing a connection to the mail server.
Here’s a basic example of connecting to an IMAP server:
1 2 3 4 5 | import javax.mail.*; // Assuming 'session' is a valid Session object Store store = session.getStore("imaps"); // Use "imap" for non-secure connections store.connect("imap.example.com", "your-username", "your-password"); |
3.4.2 Accessing the Mailbox
Once the connection is established, you can access the user’s mailbox and retrieve email messages. The Folder class represents a mailbox, and you can use it in conjunction with the Store class to manage messages.
1 2 3 4 5 | Folder inbox = store.getFolder("INBOX"); inbox.open(Folder.READ_ONLY); // Open the folder in read-only mode // Retrieve messages from the inbox Message[] messages = inbox.getMessages(); |
3.4.3 Message Retrieval and Handling
Once you have retrieved the messages, you can iterate through them and perform various actions, such as reading the content, extracting attachments, and managing flags.
1 2 3 4 5 6 7 8 9 10 11 12 | for (Message message : messages) { // Retrieve and display message details String subject = message.getSubject(); Address[] from = message.getFrom(); // Additional details can be retrieved as needed // Perform actions based on message content // ... // Mark the message as read, if necessary message.setFlag(Flags.Flag.SEEN, true); } |
3.4.4 Choosing between IMAP and POP3
The choice between IMAP and POP3 depends on the specific requirements of your application:
Use IMAP if you need to access emails from multiple devices, keep emails synchronized, and perform actions on the server without downloading the entire message.
Use POP3 if your application is designed for a single device, and you prefer downloading emails to the local machine, removing them from the server.
Let us understand this concept with a code example in the later part of this article.
4. Configuring SMTP for JavaMail
4.1 SMTP Servers and Providers
Selecting the right SMTP server is crucial for reliable email delivery. Popular providers include Gmail, Outlook, and your organization’s SMTP server. Ensure that you have the correct server address, port, and authentication details. In this article, we will be using the Gmail SMTP server.
1 2 | properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); |
4.2 Authentication
SMTP servers often require authentication to ensure that only authorized users can send emails. Provide your email address and password for authentication:
1 2 3 | properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.password", "your-email-password"); |
Note:
When using Gmail SMTP, you cannot authenticate with your regular Gmail password. Instead, you need to generate a 16-digit app password. Detailed information about this process is available at https://support.google.com/mail/answer/185833?sjid=1520987343100173206-AP.
4.3 Secure Connections (SSL/TLS)
When you’re sending emails, especially over the internet, it’s essential to encrypt the communication to safeguard the content of the messages and sensitive information such as login credentials. SSL and TLS are cryptographic protocols that provide secure communication channels, and JavaMail makes it straightforward to enable these protocols.
1 2 3 4 5 | // Enable TLS properties.put("mail.smtp.starttls.enable", "true"); // Enable SSL properties.put("mail.smtp.ssl.enable", "true"); |
But in this article, we will use TLS.
5. Sending Simple Emails
Creating a message involves specifying the sender, recipient, subject, and content. Additionally, you can customize headers and other properties.
Sending the message using the Transport class remains the same as shown in the previous section.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 | package org.example; import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.Arrays; import java.util.Properties; public class Main { public static void main(String[] args) throws MessagingException, IOException { // Creating session Session session = createSession(); // Create and configure a simple text message Message message = new MimeMessage(session); message.setSubject("Hello, JavaMail!"); message.setText("This is a test email from JavaMail."); // Now 'message' is ready to be sent Transport.send(message); System.out.println("Email sent successfully!"); } private static Session createSession() { Properties properties = new Properties(); properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.starttls.enable", "true"); return Session.getInstance(properties, new javax.mail.Authenticator() { protected javax.mail.PasswordAuthentication getPasswordAuthentication() { } }); } } |
Output:
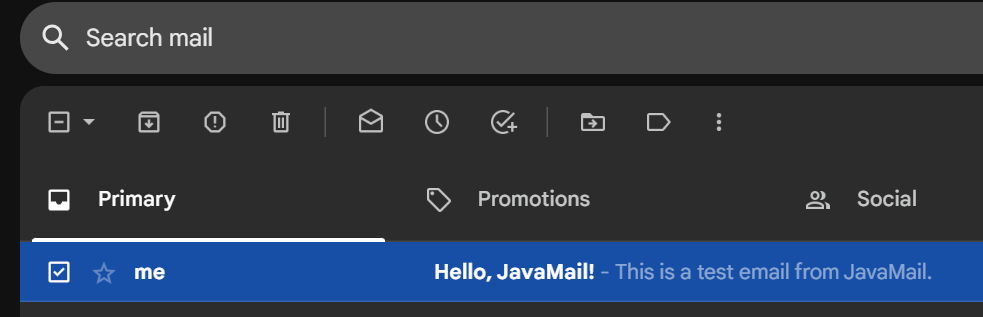
Simple Mail from Java
6. Advanced Email Features
6.1 Adding Attachments
Including attachments in your emails is a common requirement. The following code snippet demonstrates how to attach a file to your email:
- Create a Session object to encapsulate email configuration settings. The createSession() method is presumed to provide a configured session.
- Instantiate a MimeMessage object to represent the email message.
- Set sender, recipient, and subject attributes of the email message.
- Create a MimeBodyPart object to represent the text body of the email. Set the text content of the body part using setText().
- Create another MimeBodyPart to represent the attachment.Use attachFile() to attach the specified file to the body part.
- Create a MimeMultipart object to hold both the text body and the attachment.Add the created MimeBodyPart instances to the multipart container.
- Set the Multipart object as the content of the email message. This indicates that the email contains both text and an attachment.
- Use Transport.send() to send the email with the configured message.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 | package org.example; import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.Arrays; import java.util.Properties; public class Main { public static void main(String[] args) throws MessagingException, IOException { // Creating session Session session = createSession(); // Create and configure a simple text message Message message = new MimeMessage(session); mailWithAttachments(session, message); // Now 'message' is ready to be sent Transport.send(message); System.out.println("Email sent successfully!"); } private static Session createSession() { Properties properties = new Properties(); properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.starttls.enable", "true"); return Session.getInstance(properties, new javax.mail.Authenticator() { protected javax.mail.PasswordAuthentication getPasswordAuthentication() { } }); } public static void mailWithAttachments(Session session, Message message) throws MessagingException, IOException { BodyPart messageBodyPart = new MimeBodyPart(); messageBodyPart.setText("This email contains an attachment."); // Attach the file MimeBodyPart attachmentBodyPart = new MimeBodyPart(); attachmentBodyPart.attachFile("C:/Users/sai.p/Downloads/test.csv"); // Create a multipart message Multipart multipart = new MimeMultipart(); multipart.addBodyPart(messageBodyPart); multipart.addBodyPart(attachmentBodyPart); // Set the multipart as the message's content message.setContent(multipart); } } |
Output:
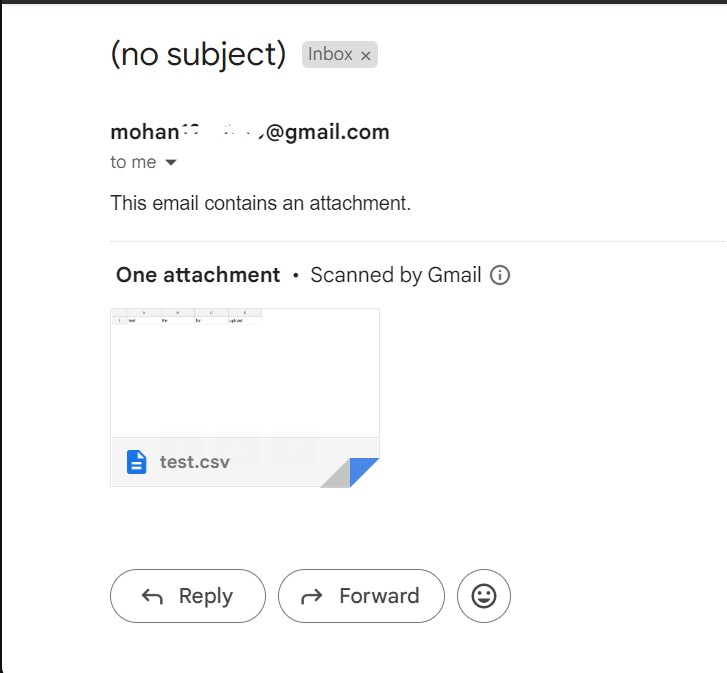
Mail with attachment (CSV file in this case)
6.2 HTML Emails
Send HTML-formatted emails for more visually appealing content.
6.2.1 Setting HTML content from a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | package org.example; import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.Arrays; import java.util.Properties; public class Main { public static void main(String[] args) throws MessagingException, IOException { // Creating session Session session = createSession(); // Create and configure a simple text message Message message = new MimeMessage(session); simpleHtmlMail(message); // Now 'message' is ready to be sent Transport.send(message); System.out.println("Email sent successfully!"); } private static Session createSession() { Properties properties = new Properties(); properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.starttls.enable", "true"); return Session.getInstance(properties, new javax.mail.Authenticator() { protected javax.mail.PasswordAuthentication getPasswordAuthentication() { } }); } public static void simpleHtmlMail(Message message) throws MessagingException, IOException { String htmlContent = "This is a simple html <b>content</b> <div style='color:red;padding:8px'>Hello Everyone!</div> "; message.setContent(htmlContent,"text/html"); } } |
Output:
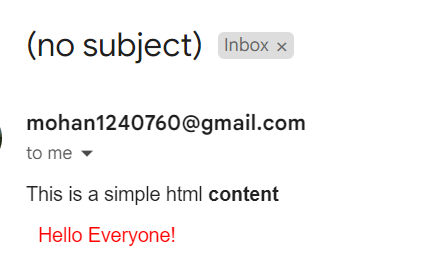
Mail with HTML Content
6.2.1 Setting HTML content from an HTML file.
- Read the contents of an HTML.
- file convert it into a string and pass it to the setContent() method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | package org.example; import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.Arrays; import java.util.Properties; public class Main { public static void main(String[] args) throws MessagingException, IOException { // Creating session Session session = createSession(); // Create and configure a simple text message Message message = new MimeMessage(session); htmlMail(message); // Now 'message' is ready to be sent Transport.send(message); System.out.println("Email sent successfully!"); } private static Session createSession() { Properties properties = new Properties(); properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.starttls.enable", "true"); return Session.getInstance(properties, new javax.mail.Authenticator() { protected javax.mail.PasswordAuthentication getPasswordAuthentication() { } }); } public static void htmlMail(Message message) throws MessagingException, IOException { String htmlContent = new String(Files.readAllBytes(Paths.get("C:/Users/sai.p/Downloads/greetings.html"))); message.setContent(htmlContent,"text/html"); } } |
Output:
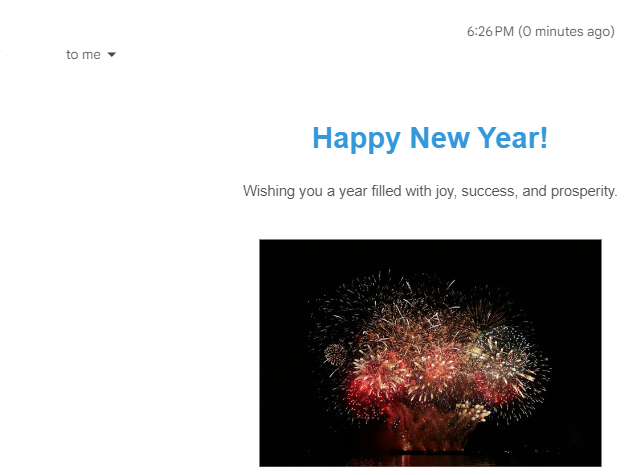
Mails sent by rendering HTML file
6.3 Retrieving mail from Mail Inbox.
- The readMails method connects to a Gmail account using IMAP and retrieves the last 10 emails from the INBOX folder.
- It connects to the Gmail IMAP server using the provided credentials.
- Accesses the INBOX folder and opens it in read-only mode.
- Retrieves the last 10 messages (or fewer if there are fewer than 10) using inbox.getMessages(inbox.getMessageCount()-10, inbox.getMessageCount()-1).
- Iterates through the retrieved messages and prints their subjects and senders.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | package org.example; import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.Arrays; import java.util.Properties; public class Main { public static void main(String[] args) throws MessagingException, IOException { // Creating session Session session = createSession(); readMails(session); System.out.println("Email sent successfully!"); } public static void readMails(Session session) throws MessagingException { Store store = session.getStore("imaps"); // Access the INBOX folder Folder inbox = store.getFolder("INBOX"); inbox.open(Folder.READ_ONLY); // Retrieve and display messages Message[] messages = inbox.getMessages(inbox.getMessageCount()-10, inbox.getMessageCount()-1); for (Message message : messages) { System.out.print("Subject: " + message.getSubject()); System.out.println(", From: " + Arrays.toString(message.getFrom())); // Additional details can be displayed } // Close the connection inbox.close(false); store.close(); } private static Session createSession() { Properties properties = new Properties(); properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.starttls.enable", "true"); return Session.getInstance(properties, new javax.mail.Authenticator() { protected javax.mail.PasswordAuthentication getPasswordAuthentication() { return new javax.mail.PasswordAuthentication("username@gmail", "password"); } }); } } |
7. Handling Email Responses
7.1 Setting Reply-To Address
Setting a “Reply-To” address in an email message is a useful feature that allows the sender to specify an alternative email address to which recipients should direct their replies. This is particularly beneficial in scenarios where the person initiating the email communication may not be the primary point of contact or where specific departments or individuals handle responses.
The setReplyTo method is used to configure the “Reply-To” address in the email message.
1 |
7.2 Handling Bounced Emails
Handling bounced emails involves monitoring and processing delivery status notifications. While this often requires additional configuration on the server side, you can set the Return-Receipt-To header to request a notification.
1 |
By configuring the return receipt, the configured mail will receive the return receipt notification.
8. Best Practices for Email Sending in Java
8.1 Error Handling and Logging
Implementing error handling ensures that your application gracefully manages exceptions. Logging mechanisms, such as Java’s built-in logging or third-party libraries like Log4j, can help track issues.
1 2 3 4 5 6 | try { // Sending email code here } catch (MessagingException e) { // Handle messaging exception e.printStackTrace(); // Log the exception } |
8.2 Performance Optimization
Optimizing email-sending performance involves minimizing network latency and resource consumption. Consider using asynchronous methods or threading for parallel email sending.
- A loop is used to send five emails concurrently, each in a separate thread.
- Inside the loop, a lambda expression creates a MimeMessage for each email, sets content, and sends it using a thread from the fixed-size thread pool.
- An ExecutorService with a fixed thread pool is employed for the concurrent execution of email-sending tasks.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | package org.example; import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeBodyPart; import javax.mail.internet.MimeMessage; import javax.mail.internet.MimeMultipart; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.Arrays; import java.util.Properties; public class Main { public static void main(String[] args) throws MessagingException, IOException { // Creating session Session session = createSession(); ExecutorService executorService = Executors.newFixedThreadPool(5); for (int i = 0; i < 5; i++) { int finalI = i; executorService.submit(() -> { try { // Create and configure a new message for each iteration Message message = new MimeMessage(session); // Set the content for this specific message message.setText("Mail number = " + finalI); // Send the message Transport.send(message); System.out.println("Email sent successfully for mail number = " + finalI); } catch (MessagingException e) { e.printStackTrace(); } }); } executorService.shutdown(); } private static Session createSession() { Properties properties = new Properties(); properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.port", "587"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.starttls.enable", "true"); return Session.getInstance(properties, new javax.mail.Authenticator() { protected javax.mail.PasswordAuthentication getPasswordAuthentication() { } }); } } |
Output:
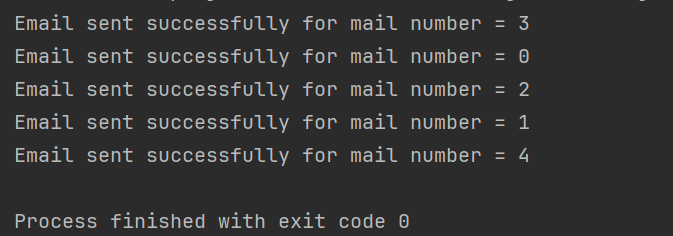
Mails sent using Multi-Threading
9. Troubleshooting Common Issues
9.1 Connection Problems
Issue: Unable to connect to the SMTP server.
Ensure that the SMTP server address, port, and connection properties are correctly configured. Additionally, check your network settings and firewall rules.
9.2 Authentication Failures
Issue: Authentication credentials are not accepted by the SMTP server.
Double-check the correctness of your email address and password. Some SMTP providers might require an application-specific password, so verify the authentication requirements.
10. Conclusion
In this comprehensive guide, we’ve covered the fundamentals of sending emails in Java applications using the JavaMail API. From setting up your environment, understanding JavaMail components, and configuring SMTP for email delivery, to implementing advanced features and handling common issues, you now have a solid foundation for incorporating robust email functionality into your Java projects.
By following best practices, testing thoroughly, and leveraging real-world examples, you can enhance user experience and streamline communication in your applications. Remember to troubleshoot any issues effectively, ensuring that your email-sending functionality is reliable and error-tolerant.
References:
- https://javaee.github.io/javamail/
- https://source.unsplash.com/ (For Images)
- https://openai.com/dall-e-2 (Thumbnail Image)
- https://deepai.org/ (Cover Image)
No comments yet